Step-by-Step Guide to Preventing JavaScript Injections
There are many different injection methods hackers use to access sensitive data. Learn about the most popular methods and how to prevent them with secure coding best practices.
Updated April 4, 2024.
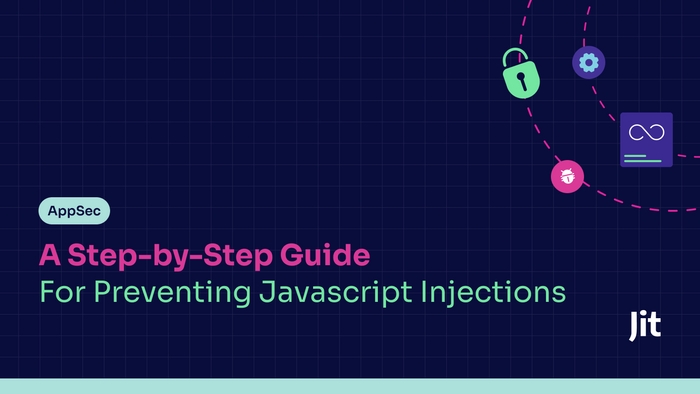
Step-by-Step Guide to Preventing JavaScript Injections
If over 40 major banks can be the target of JavaScript injection attacks, let’s be honest – so can you. In 2023, a malware campaign using this attack method affected 50,000 user sessions across more than 40 financial institutions worldwide, leaving many dev teams in pure damage-control mode.
67.9% of professional developers use JavaScript more often than any other programming language. Its popularity is understandable, given its versatile and interactive capabilities.
However, with these attacks on the rise, let's examine some practical strategies to prevent JavaScript injections so you can block malicious actors trying to access sensitive data.
JavaScript Injection Attacks: The Problem of Popularity
Secure coding practices are critically important for web applications, especially JavaScript apps. As the web's dominant scripting language, JavaScript is integral to creating engaging user interfaces and delivering dynamic content.
Yet, its widespread use also renders it a prime target for attackers aiming to exploit vulnerabilities like Text4Shell via injection attacks.
Here are some standard JavaScript injection attack methods to fortify your web applications against:
- Cross-Site Scripting (XSS) – Involves malicious scripts injected into web pages through insufficiently sanitized input fields. Attackers can then hijack user sessions, steal cookies, and capture sensitive information directly from the browser or manipulate web pages by altering the site's HTML content.
- DOM-based XSS – Occurs when attackers manipulate the Document Object Model (DOM) in the browser through JavaScript that improperly handles inputs from URLs, forms, or other sources. It is a client-side attack requiring no server interaction, allowing the execution of malicious code within the user's browser through manipulated URLs.
- Cross-Site Request Forgery (CSRF) – Attackers trick a logged-in user into unintentionally submitting a pre-crafted malicious request to a vulnerable web app. Unlike SSRF attacks, which target server-side components, CSRF targets the client side, exploiting a web application's trust in a user's browser.
- JavaScript Keylogging – Embeds a keylogger script into a web page, which captures and transmits the user’s keystrokes to a server controlled by the attacker. JavaScript Keylogging is often achieved by compromising a legitimate website or through a cross-site scripting vulnerability, allowing attackers to steal login credentials, credit card information, and other sensitive data entered into forms on the page.
- Malicious Redirection – Involves injecting JavaScript or manipulating web page redirects to send users to phishing, scam, or malware-distributing websites. Malicious redirection works through compromised advertisements, hijacked web sessions, or manipulated hyperlinks. The goal is to exploit users' trust in the original site, using it as a launch pad for phishing attacks or malware distribution.
Seven Methods for Preventing JavaScript Injections
1. Input Validation and Output Encoding
Input validation and output encoding are your first defense against various injection attacks. The goal of input validation is to guarantee that only correctly formatted data enters the system, while output encoding processes data rendered back to the user in a manner that makes the browser interpret it as data rather than executable code.
SAST and DAST tools offer a two-pronged approach to securing your web application’s data inputs and safely encoding outputs.
As an end-to-end DevSecOps orchestration platform, Jit offers out-of-the-box coverage for application security tools like SAST, DAST, and secrets detection across repos in a matter of minutes.
Under the hood Jit leverages tools like Semgrep to scan your source code to identify unsafe coding patterns and potential injection points early in the development cycle. Similarly, Jit uses ZAP to dynamically test for vulnerabilities that become apparent only during execution, such as those exploited by JavaScript injection attacks.
For example, as you create new PRs, Jit will trigger a security finding if you are improperly using methods like innerHTML or outerHTML, which allows unsanitized user-controlled data to be treated as HTML, opening up your application to XSS attacks.
2. Content Security Policy (CSP)
By defining trustworthy content sources, a Content Security Policy (CSP) helps limit the risk of executing malicious scripts that attackers embed in web pages.
To implement CSP, you must configure the web server to send the Content-Security-Policy HTTP header. This header allows the specification of directives that define the sources from which different types of content can be loaded.
For example, to prevent inline scripts from running and restrict scripts to those hosted on the same origin, your policy would be configured as follows:
Content-Security-Policy: script-src 'self';
For more granular control, developers can specify different sources for different types of content, such as images, fonts, and scripts. It's also possible to allow scripts from specific trusted domains while blocking all others that could open the door to JavaScript injections.
It is best to start with a restrictive policy and gradually relax it as necessary rather than starting with a permissive policy and tightening it.
3. Use HTTPS
Encrypt your data in transit by switching to HTTPS, shielding it from Man-in-the-Middle (MitM) attacks where bad actors might intercept and tamper with the information.
You'll need a digital certificate from a Certificate Authority (CA) to activate HTTPS. Start by generating a private key and a Certificate Signing Request (CSR), then submit your CSR to a CA.
Once you receive your SSL/TLS certificate, configure your web server to use it. Modern web servers like Apache, Nginx, and IIS have straightforward configurations for SSL/TLS that guide through the process.
Boost your site's security further with HTTP Strict Transport Security (HSTS). HSTS tells browsers only to use HTTPS connections – preventing downgrade attacks where an attacker might try to force a connection to revert to HTTP. You can implement HSTS by adding the following header to your web server configuration:
Header always set Strict-Transport-Security "max-age=31536000; includeSubDomains"
4. Parameterized Statements for Database Queries
To shield your database from SQL injection, lean on prepared statements with parameterized queries.
Instead of dropping user-entered data straight into your queries, use placeholders. Then, feed the actual data to the query separately. This strategy ensures the database sees the input as just data, stripping away its potential to execute as code.
Make parameterized queries your go-to by tapping into the prepared statement functionalities provided by your database management system (DBMS). For example, in a PHP environment using MySQL, instead of directly inserting user input into a SQL query, you would use prepared statements with placeholders:
$stmt = $conn->prepare("SELECT * FROM users WHERE email = ?");
$stmt->bind_param("s", $userEmail);
$stmt->execute();
$result = $stmt->get_result(); Here, ? is a placeholder for the user input, and $userEmail is a variable containing the user input. The bind_param method binds the user input to the placeholder, treating it as a string.
5. Regular Security Audits and Penetration Testing
Conduct regular security audits and penetration testing to uncover and address JavaScript vulnerabilities. Leverage OWASP ZAP, a powerful penetration testing tool, to simulate real attack injection scenarios on your application.
Jit amplifies the value of these security practices by offering a streamlined approach to identifying, assessing, and mitigating security risks. With Jit, you can easily deploy ZAP with the configuration wizard, rather than figuring it out and testing it yourself.
Jit’s platform enhances traditional penetration testing methods with automated tools and workflows, ensuring that security testing is not only thorough but also efficiently integrated into your software development lifecycle.
6. Client-Side Validation
Client-side validation is a type of input validation used to check your user's input's correctness, meaningfulness, and security before it hits your server.
Begin by cataloging every place in your application where users can input data – this spans text inputs, radio buttons, checkboxes, and select dropdowns.
You need to pin down the validation rules for each of these fields. What data type are you expecting? What format should the data follow? How long can the input be, and are there specific value ranges you're looking for?
Use JavaScript to create functions that check whether the user input meets the specified criteria. Design your validation to give instant feedback to the user. This feedback could be error messages displayed next to the input field or turning off the submit button until all inputs are valid.
Here's a simple example of validating an email address using JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Email Validation Example</title>
<script>
function validateEmail() {
var emailInput = document.getElementById('email').value;
var regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (regex.test(emailInput)) {
document.getElementById('emailFeedback').style.color = 'green';
document.getElementById('emailFeedback').innerHTML = 'Valid email address.';
} else {
document.getElementById('emailFeedback').style.color = 'red';
document.getElementById('emailFeedback').innerHTML = 'Invalid email address.';
}
}
</script>
</head>
<body>
<label for="email">Email:</label>
<input type="text" id="email" onkeyup="validateEmail()">
<p id="emailFeedback"></p>
</body>
</html> 7. Use Security Headers
Take charge of how browsers interact with your site's content by utilizing security headers. Your main objective here is to dictate what resources the browser can load.
Consider implementing these headers:
X-Content-Type-Options – Activate this header with the nosniff option to stop the browser from MIME type sniffing. This precaution prevents the browser from treating non-script files as scripts, lowering the risk of injection attacks. Adjust your server settings to include X-Content-Type-Options: nosniff in all HTTP responses.
- X-Frame-Options – Deploy this header to shield against clickjacking attacks. By setting X-Frame-Options: DENY or X-Frame-Options: SAMEORIGIN, you limit or entirely block other sites from framing your content. This secures your site against certain types of JavaScript injection attacks and unauthorized content embedding.
Fighting JavaScript Injections With Proactive Measures
The battle against JavaScript injections demands a proactive, layered defense strategy. By embracing thorough validation, encoding, and the strategic use of security tools and features, you fortify your web applications against these pervasive threats.
Jit's DevSecOps platform can simplify and enhance your approach to hardening your web apps against injection attacks.
It’s designed to integrate a suite of security testing and control tools into your SDLC to detect vulnerabilities early in the development process. With Jit, your development team can efficiently identify vulnerabilities, including those leading to JavaScript injection attacks, well before they escalate into security breaches. Explore more here.